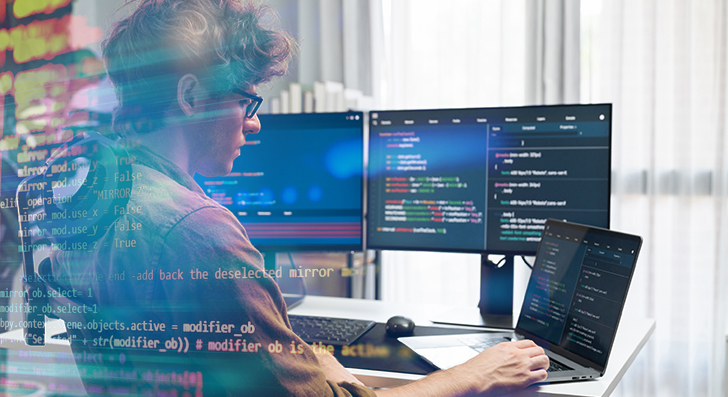
Scalability suggests your software can tackle expansion—a lot more customers, more facts, plus much more site visitors—with out breaking. To be a developer, making with scalability in mind will save time and anxiety afterwards. Listed here’s a clear and realistic guidebook to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability is not something you bolt on later on—it ought to be element within your prepare from the start. Several purposes fall short every time they expand speedy due to the fact the first design and style can’t tackle the extra load. Being a developer, you need to Consider early regarding how your program will behave stressed.
Start by planning your architecture to generally be flexible. Prevent monolithic codebases where almost everything is tightly related. Instead, use modular layout or microservices. These patterns break your application into lesser, independent elements. Just about every module or service can scale on its own without having impacting The entire technique.
Also, give thought to your database from day a single. Will it need to have to take care of one million users or perhaps 100? Pick the right kind—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them but.
A further vital point is to avoid hardcoding assumptions. Don’t create code that only operates below existing problems. Think of what would transpire if your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design designs that assist scaling, like concept queues or occasion-pushed programs. These support your app deal with much more requests with out getting overloaded.
When you Establish with scalability in your mind, you are not just planning for achievement—you are decreasing future problems. A very well-planned program is easier to take care of, adapt, and mature. It’s superior to get ready early than to rebuild later.
Use the Right Databases
Selecting the correct databases can be a crucial A part of developing scalable purposes. Not all databases are created the identical, and using the Erroneous one can gradual you down as well as trigger failures as your application grows.
Commence by knowing your information. Can it be very structured, like rows inside of a desk? If yes, a relational databases like PostgreSQL or MySQL is a good healthy. These are generally robust with interactions, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle far more visitors and facts.
In case your data is much more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured data and will scale horizontally much more simply.
Also, consider your go through and produce patterns. Do you think you're accomplishing plenty of reads with less writes? Use caching and skim replicas. Have you been managing a heavy create load? Investigate databases which can deal with substantial produce throughput, or even occasion-based mostly facts storage methods like Apache Kafka (for short term facts streams).
It’s also good to think ahead. You may not want Innovative scaling capabilities now, but deciding on a databases that supports them indicates you gained’t want to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your facts based upon your obtain styles. And normally keep track of database overall performance as you develop.
In brief, the proper database depends on your application’s composition, velocity desires, And exactly how you hope it to mature. Choose time to select correctly—it’ll preserve plenty of difficulty later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, every single tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Make productive logic from the start.
Commence by writing clean up, basic code. Stay away from repeating logic and remove just about anything unneeded. Don’t choose the most elaborate Option if an easy one will work. Keep the features short, centered, and easy to check. Use profiling resources to find bottlenecks—destinations in which your code usually takes way too lengthy to operate or works by using a lot of memory.
Next, have a look at your database queries. These typically slow factors down more than the code by itself. Make sure Every single query only asks for the information you actually will need. Steer clear of Pick out *, which fetches every thing, and as a substitute choose distinct fields. Use indexes to hurry up lookups. And stay away from executing too many joins, Specially throughout big tables.
For those who recognize the exact same data getting asked for again and again, use caching. Retailer the final results quickly utilizing equipment like Redis or Memcached this means you don’t need to repeat high priced functions.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app a lot more successful.
Make sure to exam with large datasets. Code and queries that operate great with a hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable applications are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These ways assist your application remain smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and much more site visitors. If every little thing goes by means of a single server, it can promptly turn into a bottleneck. That’s the place load balancing and caching are available in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of one server doing many of the get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-centered solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused rapidly. When users ask for exactly the same information and facts yet again—like a product web site or possibly a profile—you don’t have to fetch it within the databases each and every time. You can provide it from the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly obtain.
2. Shopper-side caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app far more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when information does transform.
In short, load balancing and caching are basic but impressive resources. Jointly, they assist your app take care of extra customers, keep speedy, and recover from troubles. If you propose to grow, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you need resources that allow your application mature easily. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling A lot smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to get components or guess long run potential. When targeted visitors improves, you can add much more resources with just a few clicks or immediately employing car-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your application in lieu of taking care of infrastructure.
Containers are A different essential Device. A container packages your application and every thing it needs to operate—code, libraries, options—into a single unit. This can make it effortless to move your application involving environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application works by using several containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it mechanically.
Containers also allow it to be straightforward to individual elements of your application into providers. You can update or scale sections independently, which can be perfect for functionality and reliability.
In short, employing cloud and container tools suggests you'll be able to scale speedy, deploy very easily, and Get better swiftly when problems come about. If you want your app to mature without having limits, commence applying these resources early. They help save time, decrease chance, and allow you to continue to be focused on creating, not correcting.
Monitor Almost everything
For those who don’t keep track of your software, you received’t know when things go Improper. Checking helps the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a essential Portion of making scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just keep track of your servers—keep track of your app also. Control just how long it will require for people to load internet pages, how often errors occur, and exactly where they more info happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential issues. As an example, Should your response time goes above a limit or simply a company goes down, you'll want to get notified straight away. This can help you deal with troubles rapidly, usually in advance of end users even recognize.
Monitoring is usually handy if you make alterations. Should you deploy a brand new aspect and find out a spike in mistakes or slowdowns, you are able to roll it again in advance of it triggers genuine destruction.
As your application grows, visitors and details raise. Without having checking, you’ll miss out on signs of issues right up until it’s way too late. But with the correct applications in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about comprehending your procedure and ensuring it really works effectively, even stressed.
Last Views
Scalability isn’t just for major businesses. Even compact apps will need a strong foundation. By building thoroughly, optimizing wisely, and utilizing the ideal equipment, you could Construct applications that grow easily without the need of breaking under pressure. Start off compact, Feel major, and build sensible.